GDE Overview
Mathwrist User Guide Series
Abstract
This document gives a high level overview of using Mathwrist’s Graphical Debugging Extension (GDE) tool for scientific visualization in Microsoft Visual Studio IDE while developing C++ numerical programs. More detailed information can be found in subsequent user guide series by feature chapters.
1 Introduction
Mathwrist’s Graphical Debugging Extension (GDE) product is a C++ debugging extension tool for Microsoft Visual Studio 2022 community, professional and enterprise editions. It allows scientists and engineers to directly view data and math objects while performing C++ numerical programming or prototyping tasks. By rendering scientific plots inside the Visual Studio IDE, users can obtain immediate visual feedback along the course of developing and refining their algorithms or mathematical models.
GDE is published to Visual Studio Extension Marketplace. Users can download the software from the marketplace, or directly install it under Visual Studio menu ExtensionsManage Extensions and search for “GDE” from the list of online extensions. Once installed to Visual Studio as illustrated in Figure 1, GDE can be used free of charge with limited features. In order to run GDE of full features, a valid license file is required.
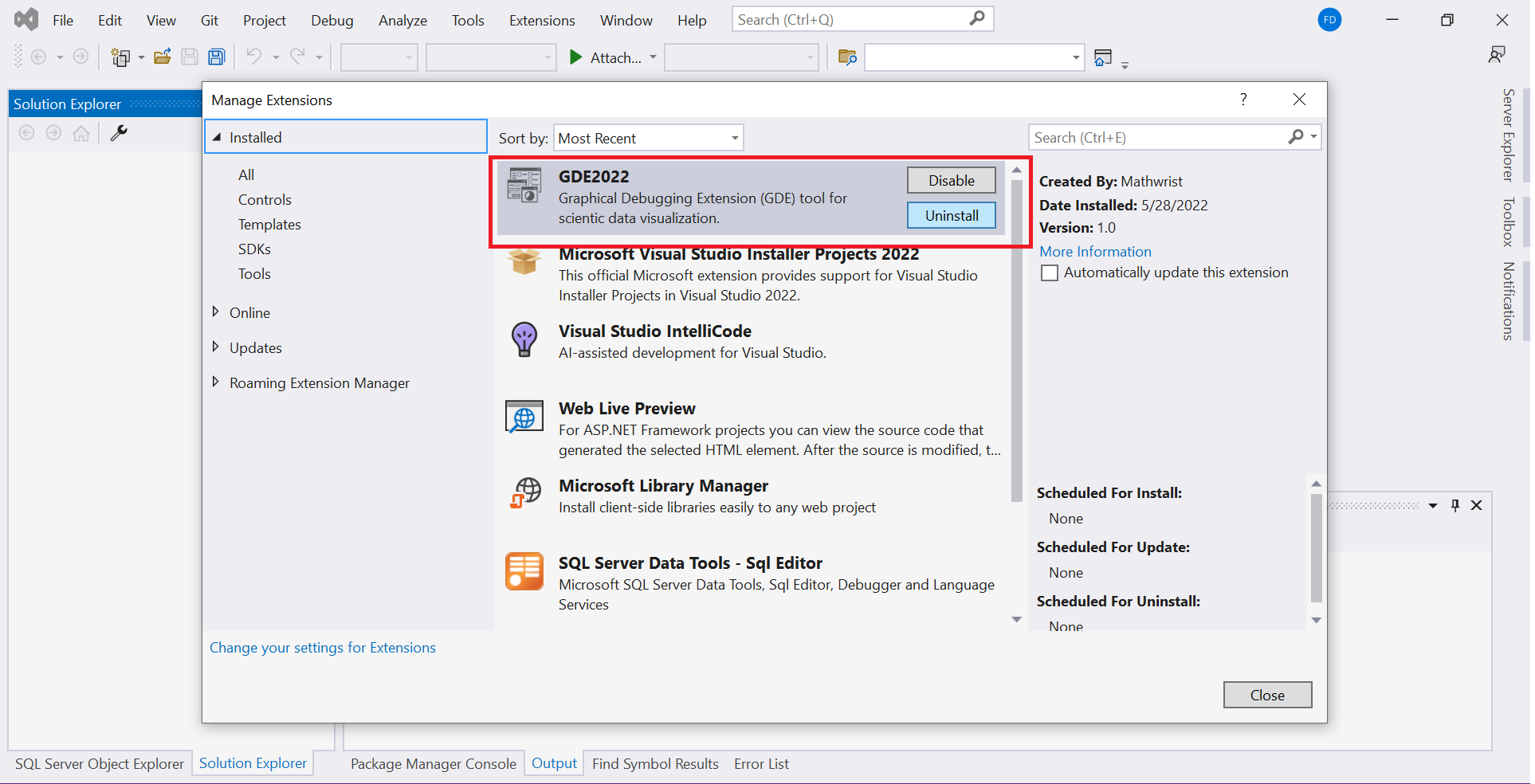
Mathwrist uses a standard Windows software installer to deploy a license activation application and its C++ numerical programming library (NPL) product to user’s desktop. GDE and NPL use the same license activation application to generate a node-locked license file. This software installer will be available for download from Mathwrist website Pricing Free Get started under either NPL or GDE product. After a purchase order is completed on Mathwrist website, the installer link will also be available from user’s order history page. Please refer to NPL user guide for the details of license activation.
2 Plottable Data Types
By default, C++ native double static array and std::vectordouble are automatically supported for plotting as “vector” data. This is a free feature to all users. Full featured software licenses will allow users to import user-defined “vector” and “matrix” C++ data types to GDE via a plottable specification json file. Most importantly, many built-in block data types defined in Mathwrist’s C++ Numerical Programming Library (NPL) are registered in GDE as plottables. NPL users have the advantage of working in an integrated environment equipped with numerical algorithms and visualization tools.
Let be the variable name of a plottable object in a C++ debugging program. By right clicking the variable name at a break point where object is in scope, GDE dynamically adds a “plot ” command to Visual Studio debugging context menu. Clicking this context menu command will either directly display object in a GDE plot window or allow users to choose relevant plotting format from a popup window. Users can further customize the display settings of the plot window and manage plottable objects in various plot sessions.
3 Plot Window
After a successful installation of the GDE package, GDE will register a menu item to Visual Studio IDE, under the path Views Other Windows GDE Plot. This is illustrated by the red arrow in figure 2. Clicking this menu item will bring up the GDE plot window to the front of IDE.
Like other Visual Studio tool windows, GDE plot window can be docked within the standard IDE layout panel, or can be undocked as a floating window at a convenient location on the screen. In the demo example of figure 3, we have docked the GDE plot window side by side to the source code editor, tabbed together with other Visual Studio debugging windows, i.e. callstack, output windows.
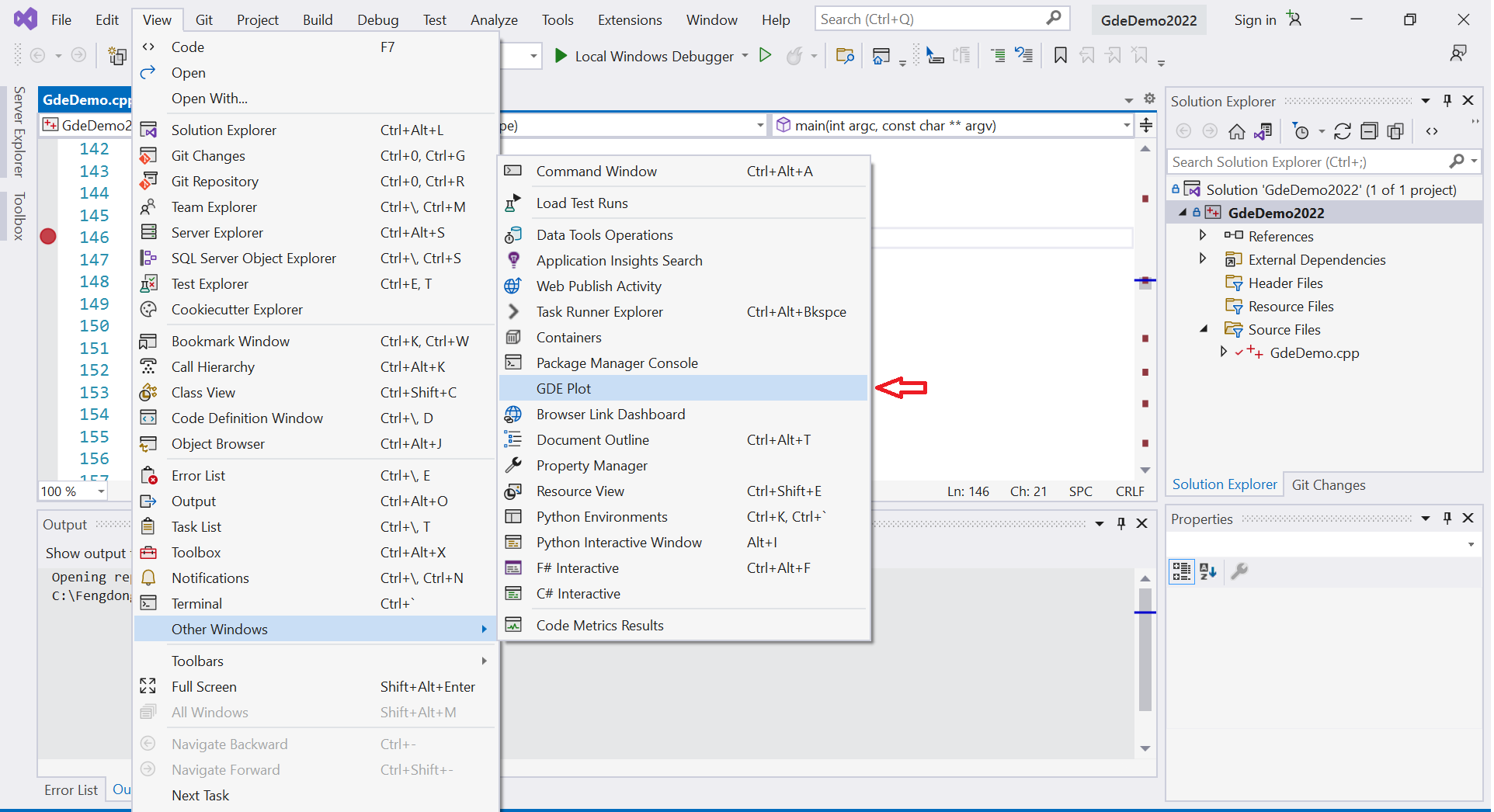
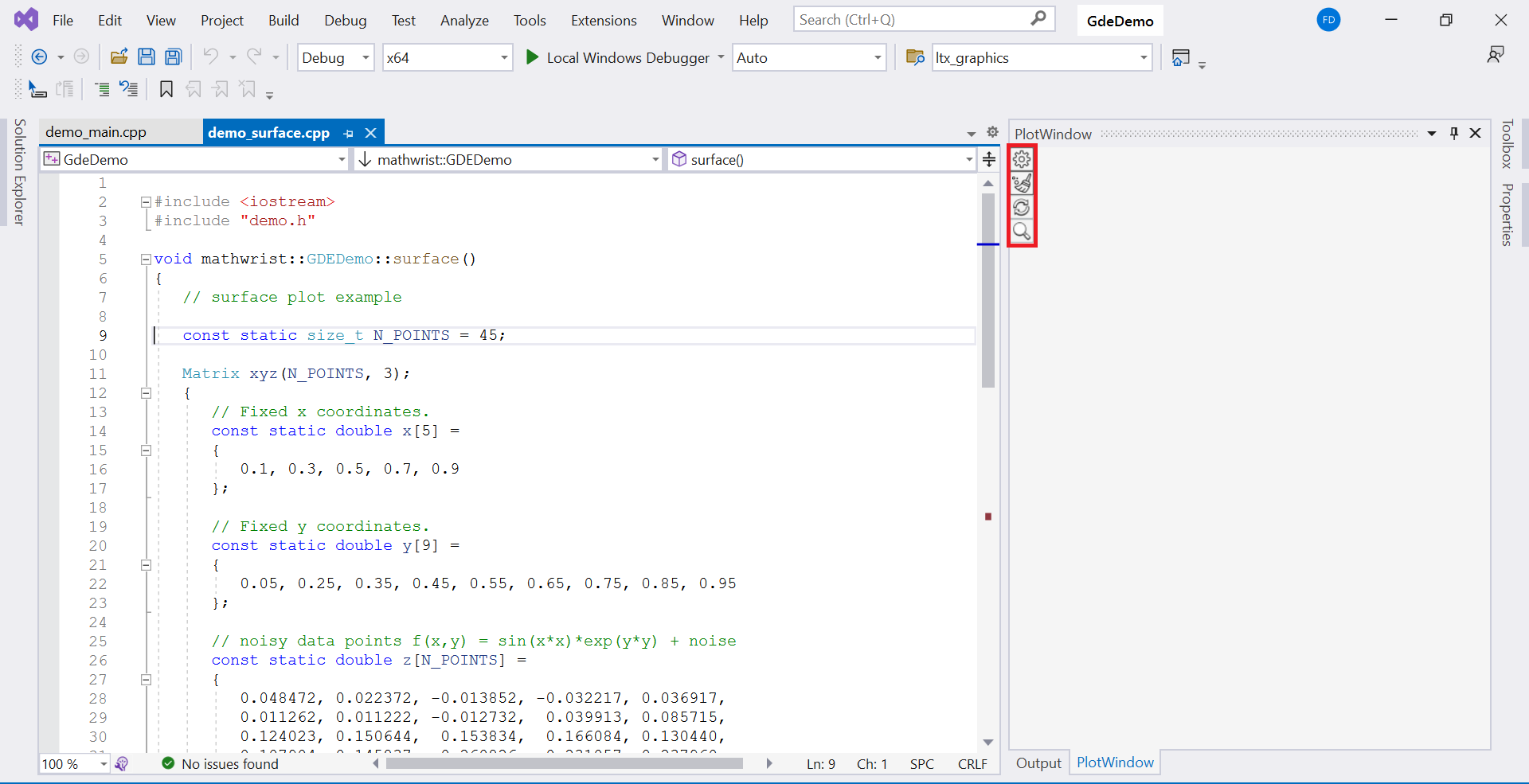
4 Settings
The red rectangle area in figure 3 is a group of GDE control buttons, ,
,
and
from top to bottom respectively. Moving the mouse over these buttons, we can see a tool tip text block emerging to indicate the purpose of these buttons, “Documentation”, “Plot Settings”, “Clear Plot” and “3-d Zooming”. Initially, “3-d Zooming” button is disabled in gray color. When a 3-d plottable object is displayed in the plot window, this button will then be enabled to support zooming 3-d objects.
The “Plot Settings” button is used to customize display control parameters. It opens up a plot settings window illustrated in example figure 4. This settings window has 3 tabs “Curve Session”, ”Surface Session” and “Data Session” marked by a green rectangle in figure 4. Each tab allows users to set display control parameters and manage plottable components in the corresponding session.
When a plottable object is sent to the plot window for display, depending on the nature of this object, it will be cached in one of these three sessions. We shall call the session that is currently displayed in the plot window the “Active Session”. When the “Clear Plot” button is clicked, the display window and all components of the active session are cleared.
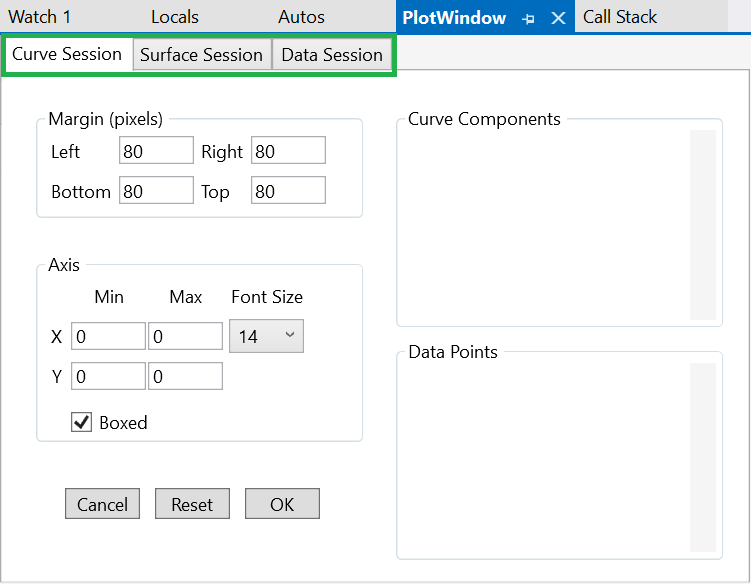
5 Object Bookkeeping
Plottable objects are bookkept by their unique names in each session. Within the same session, if two objects with the same variable name are added, the latter one always replaces the first object. In other words, one session only caches a unique name in its plottable component list.
What could be interesting to users is that, if the debuggee program stops at a break point where an object of name is in scope in the current debugging call stack, further if this object has the same data type as the plottable component represented by variable in the active session, then this plottable component will be automatically updated by object in the current stack. This feature could be very useful when we want to visualize how evolves, i.e. over iterations.
It is important to keep in wind that at each break point, the plottable name always represents the current live object in the current debugging call stack. All plottable components in a session are cached until they are explicitly deleted by the user. They are automatically updated when i) the debuggee stops at a breakpoint; and ii) their names and types match the live objects in the current call stack. When the debuggee process terminates, all plottable objects are still saved in relevant GDE sessions.
6 Session Management
As discussed earlier, clicking the “Plot Settings” button opens up a plot settings window that has “Curve”, “Surface” and “Data” three session management tabs. A full picture of this plot setting window within Visual Studio IDE is illustrated in figure 5. The left part of each tab, marked in a blue box area, contains controls that allow users to customize the display of that session. The right part of the tab, marked in a red box, contains a list of plottable components that have been added to the session. Right clicking a component name will trigger a context menu that allows users to delete this component from the current session, or display the component in applicable alternative plot types. We will discuss more session-specific details in subsequent GDE user guide series.
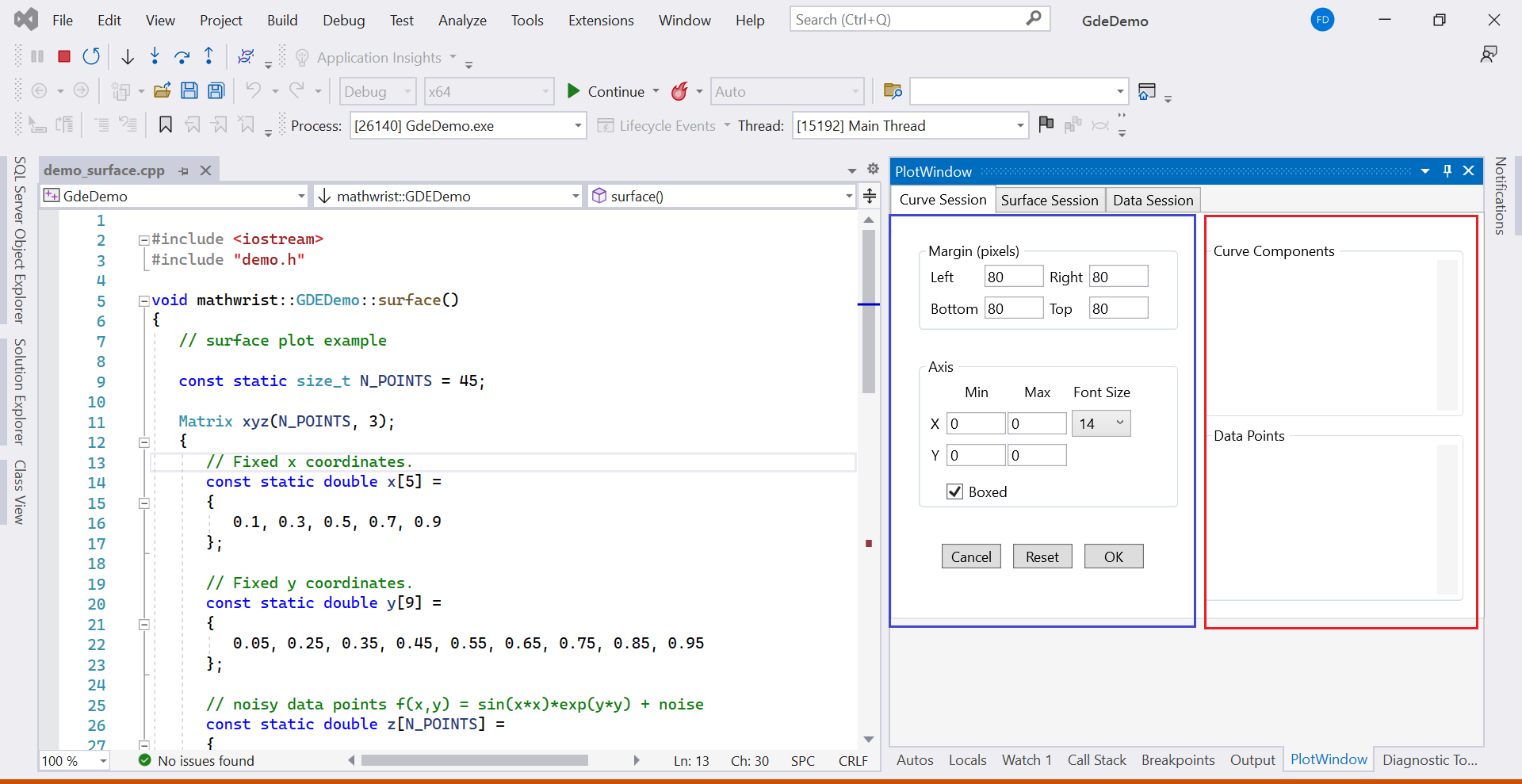
7 Plottable Specification File
By default, std::vectordouble and C++ native static array, i.e. double x[100], are recognized by GDE as vector plottables. Users may have their own implementation of vectors and matrices. It is possible to register user defined data types to GDE via a specification json file.
Each time when GDE is loaded to Visual Studio, it searches for a json file “mathwrist.gde.spec.json” under the Windows user profile directory. This directory has environment variable name “USERPROFILE” in Windows 10 and 11, which points to “C:Usersxxx” directory where “xxx” is the user name. If the plottable specification file is found present in the user profile directory, GDE will import these user defined data types as plottable types.
7.1 Vector Types
In this section and the next “Matrix Types” section, we will illustrate how to specify a user-defined data type by examples. First, please find the following C++ code Listing 1 from the demo.h header file in the “GdeDemo” project that we shipped together with GDE installation.
This simple buffer class stores dynamically allocated memory to its class member m_data and the number of elements to member m_size. The corresponding json specification of this buffer class starts from line 3 to line 7 in code Listing 2. Keyword “Vectors” at line 2 tells GDE that this specification block contains a list of vector types. Each open/close bracket {…} in between [ and ] is a single vector-type specification.
The keyword “TypeName” at line 4 indicates that the exported vector type is called mathwrist::GDEDemo::Buffer. Note that namespace “mathwrist” and outer class name “GDEDemo” are all required in order to correctly refer to the given type. Keyword “MemberSize” at line 5 indicates the vector size is stored in class/struct data member m_size. And finally keyword “MemberPointer” at line 6 indicates the vector data storage pointer is m_data.
Alternatively, a vector type specification can provide the class/struct member name of the one-beyond-last element instead of specifying the vector size. This is illustrated from line 8 to line 12 for std::vectordouble. Here, “MemberPointer” is still the starting memory address of the std vector, which is _Mypair._Myval2._Myfirst in Microsoft’s std::vector implementation. Keyword “MemberLast” indicates that the memory address of the one-beyond-last element can be obtained from class/struct data member _Mypair._Myval2._Mylast.
7.2 Matrix Types
In the same demo.h header file, we also provided a C++ matrix class definition that uses std::vector as data storage. The complete code example is included in Listing 3. Its json specification is given in Listing 4.
Here, keyword “ConsecutiveMatrices” tells GDE that this specification block is to export matrix types that use consecutive memory storage. We have only one type in this block, which is mathwrist::GDEDemo::Matrix following the keyword “TypeName” at line 3.
Line 4 and 5 indicate the number of rows and columns are stored in class/struct data member m_rows and m_cols, after keywords “MemberRowSize” and “MemberColumnSize” respectively. At line 6, keyword “MemberPointer” tells GDE that the memory address of matrix element storage can be obtained by following the chain of data members m_storage._Mypair._Myval2._Myfirst.
Lastly, keyword “RowMajor” at line 7 is used to specify whether the matrix uses row-major or column-major memory storage. In this example, we tell GDE the row-major flag is stored in class/struct member m_row_wise. If this line is missing, by default we interpret the matrix uses row-major storage. Alternatively, users can specify key-value pairs “RowMajor”: “TRUE” or “RowMajor”: “FALSE” for matrix types that consistently use row-major or column-major storage.