GDE Data Plots
Mathwrist User Guide Series
Abstract
This document is a user guide of using Mathwrist’s Graphical Debugging Extension (GDE) tool to visualize raw data in Microsoft Visual Studio IDE when debugging a C++ program. For an overview of GDE, please refer to our “GDE Overview” user guide.
1 Introduction
In this document, we focus on GDE “Data Session” and give details on how to use GDE to plot raw data of C++ standard types, Mathwrist’s Numerical Programming Library (NPL) data types and user specified plottable types that are defined in a json file. It is highly recommended to first read our “GDE Overview” user guide to have a high level understanding of GDE. The details of how to export user defined data types to GDE via a json specification file are covered in our “GDE Overview” user guide as well.
In general, we treat raw data plottables as either vectors or matrices. Vector data includes C++ static array, std::vectordouble, user defined vector data types, and 1-row or 1-column matrices. Objects of vector data type can be plotted as data series or histograms. Multi-row and multi-column matrix objects can be plotted as row data series, column data series, histogram and heat map. Users have opportunities to choose the plot format in relevant contexts.
2 Walkthrough Example
All code examples listed here are part of the “GdeDemo” Visual Studio project that is shipped together with GDE installation.
2.1 Vector Objects
The sample code Listing 1 demonstrates plotting vector data as series and histograms. To run this example, we set command line argument –series in debugging property of “GdeDemo” project. First, from line 11 to line 22, we read a series of signal data from a text file and store it in the standard std::vector variable signals. Since std::vectordouble is a standard type supported by GDE internally, variable signals is recognized as a built-in plottable data type. The sequence of steps to display this raw data object signals is the following:
-
1.
When the debuggee process stops at a breakpoint line 27, right clicking the variable name signals brings the debugging context menu to the front of IDE in Figure 1.
-
2.
Click the “Plot signals” command from the context menu triggers a data plot choice popup window in Figure 2. This popup allows users to choose either data series plot or histogram plot.
-
3.
Check the “Data Series” radio button and click OK in the popup window. The data series plot is displayed in the plot window in Figure 3.
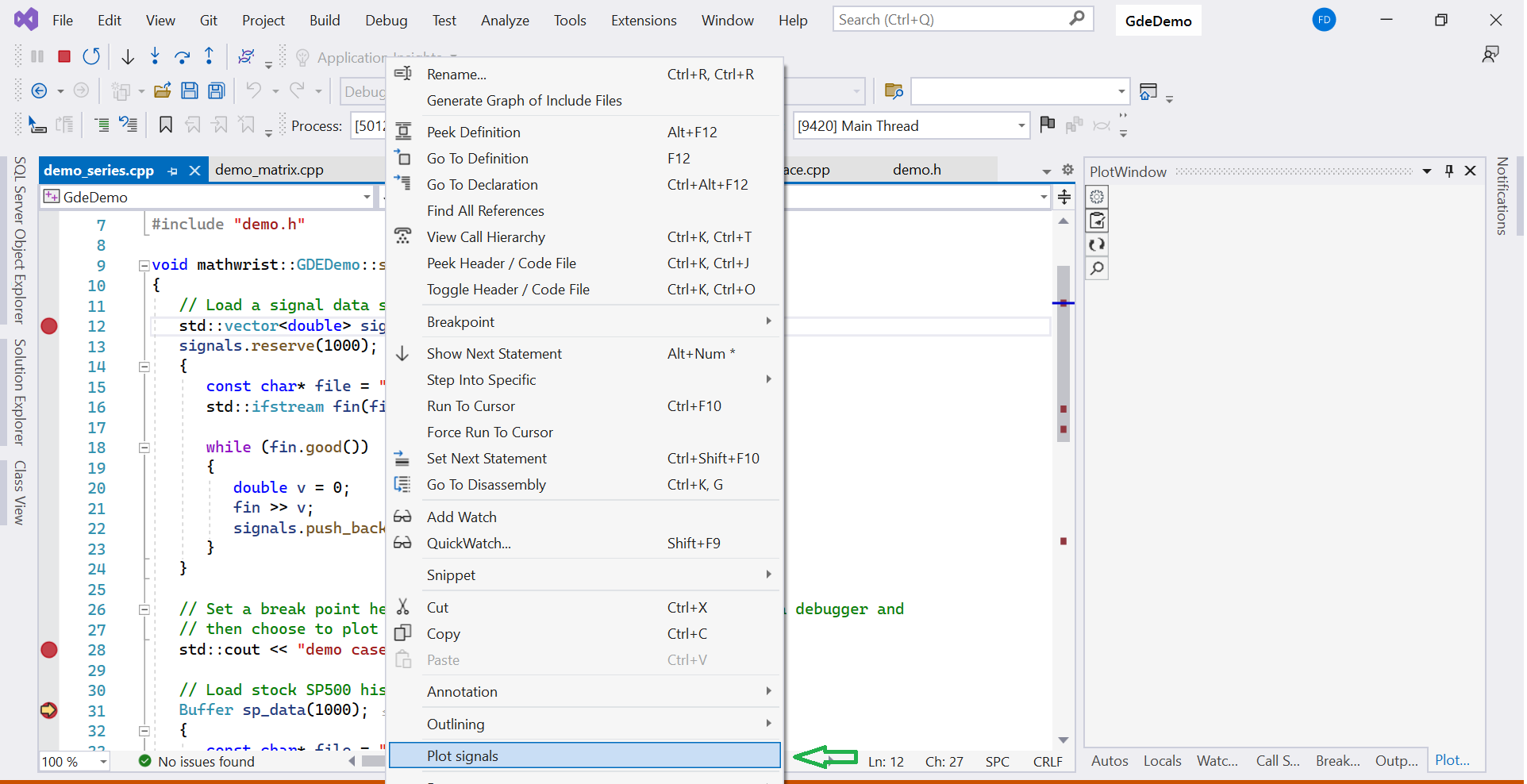
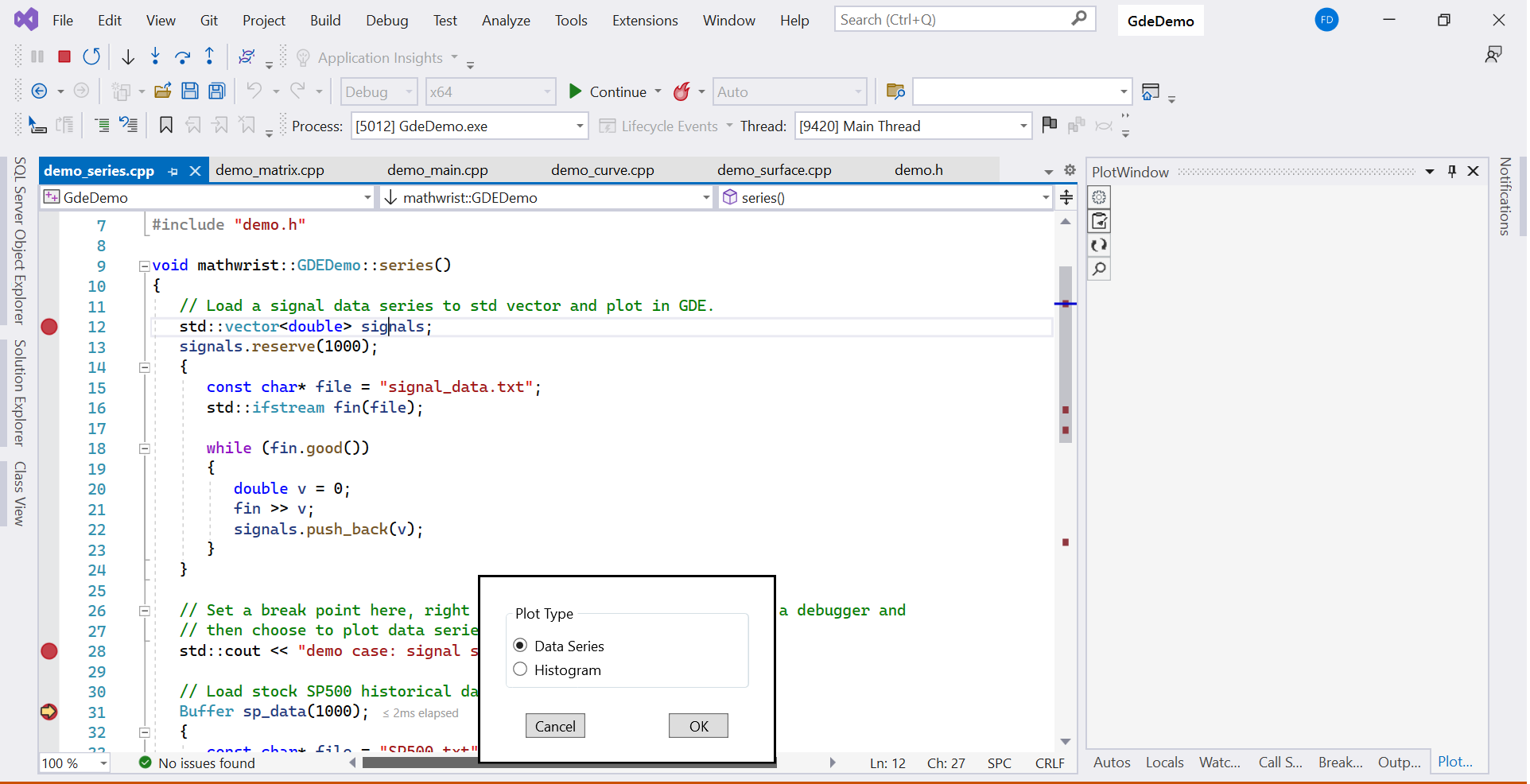
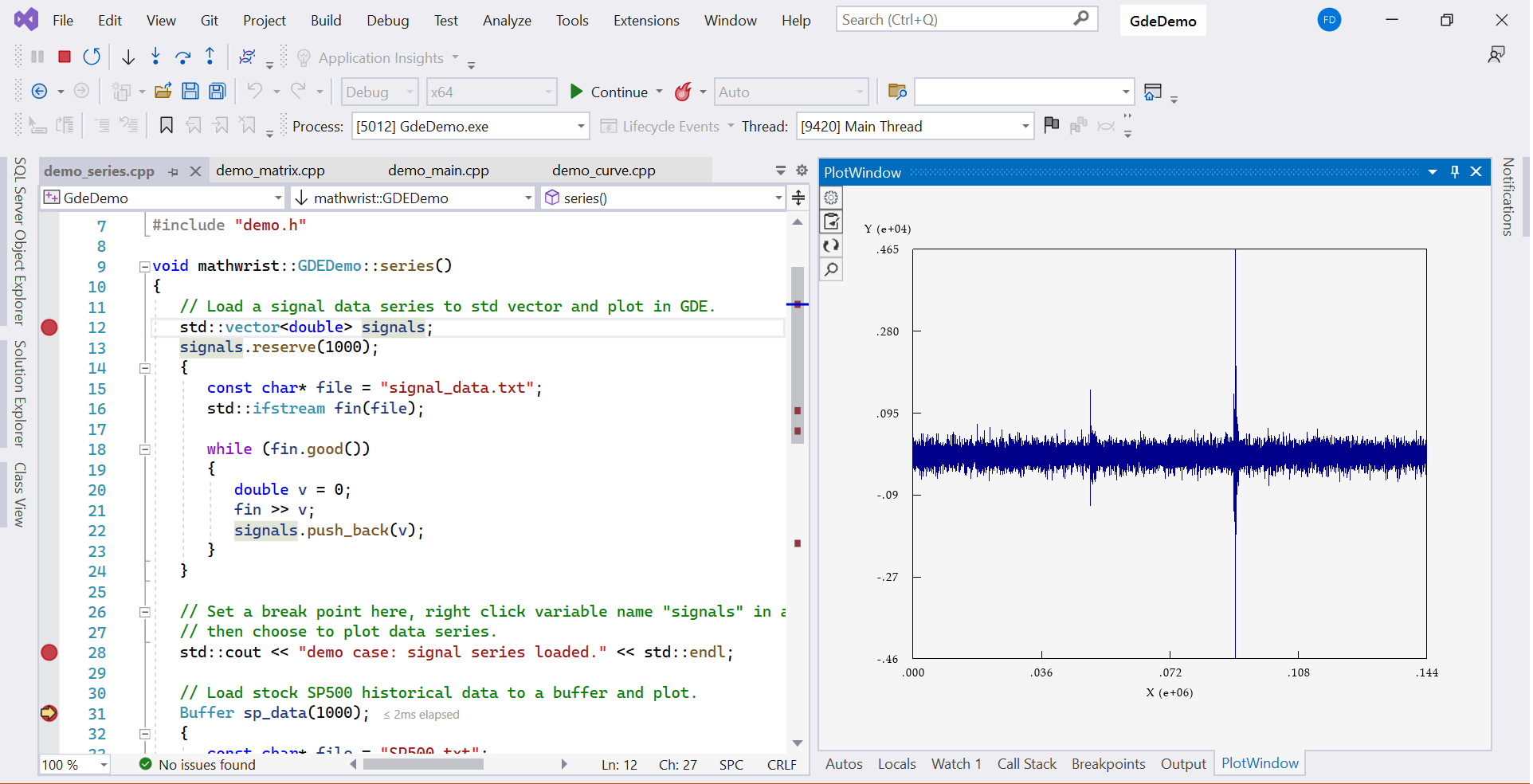
Next, from line 30 to 39, we read stock market SP500 index historical data from a text file and store it to a user-defined Buffer data structure with variable name sp_data. As we discussed in “GDE Overview” user guide, by supplying a “mathwrist.gde.spec.json” plottable specification json file and exporting user-defined type Buffer to GDE, variable sp_data at line 31 is also recognized as a plottable object. At breakpoint point line 43, one can repeat the same steps as we plot signals except that now the context menu item becomes to “Plot sp_returns”.
Lastly, we compute the daily log return of SP500 data and store it to user-defined Matrix type with variable sp_returns from line 43 to 46. Matrix is also exported to GDE through the specification json file. Note that matrix object sp_return has only 1 column, hence is treated as a vector. Right clicking variable name sp_returns when the debugger stops at break point line 51, we can choose “Plot sp_return” as a histogram plot as illustrated in Figure 4.
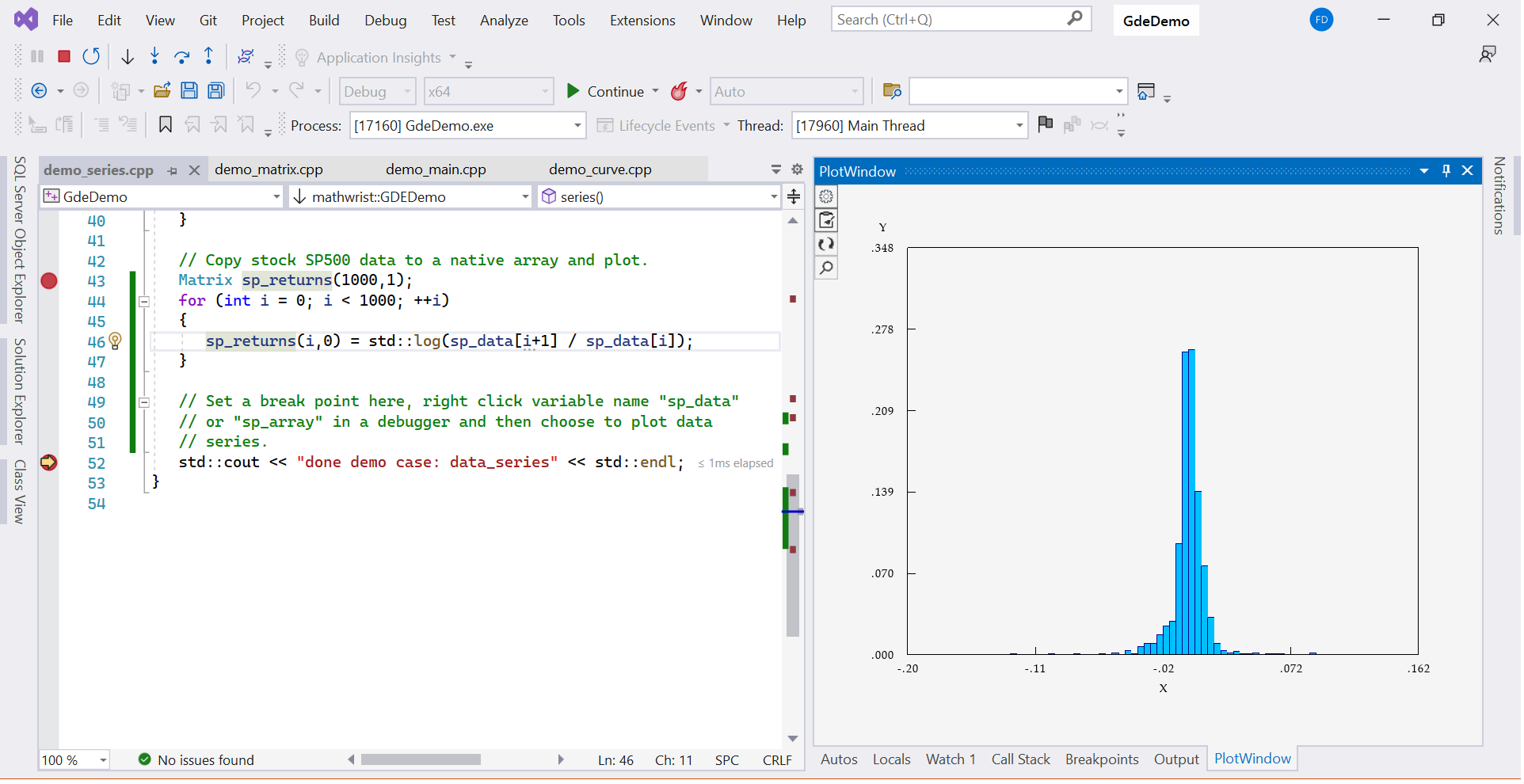
2.2 Matrix Objects
Sample code Listing 2 illustrates a multi-row and multi-column matrix object can be plotted as data series, histograms and heat maps. This example requires to set command line argument –matrix to “GdeDemo” project.
Between line 11 and 25, we populate a square matrix with standard Gaussian density. When the demo debug program stops at break point line 30, right clicking on variable name and choose “Plot ” from Visual Studio debugging context menu. A popup window Figure 5 provides choices to plot the rows or columns of matrix as data series, or alternatively plot all elements to a histogram or a heat map.
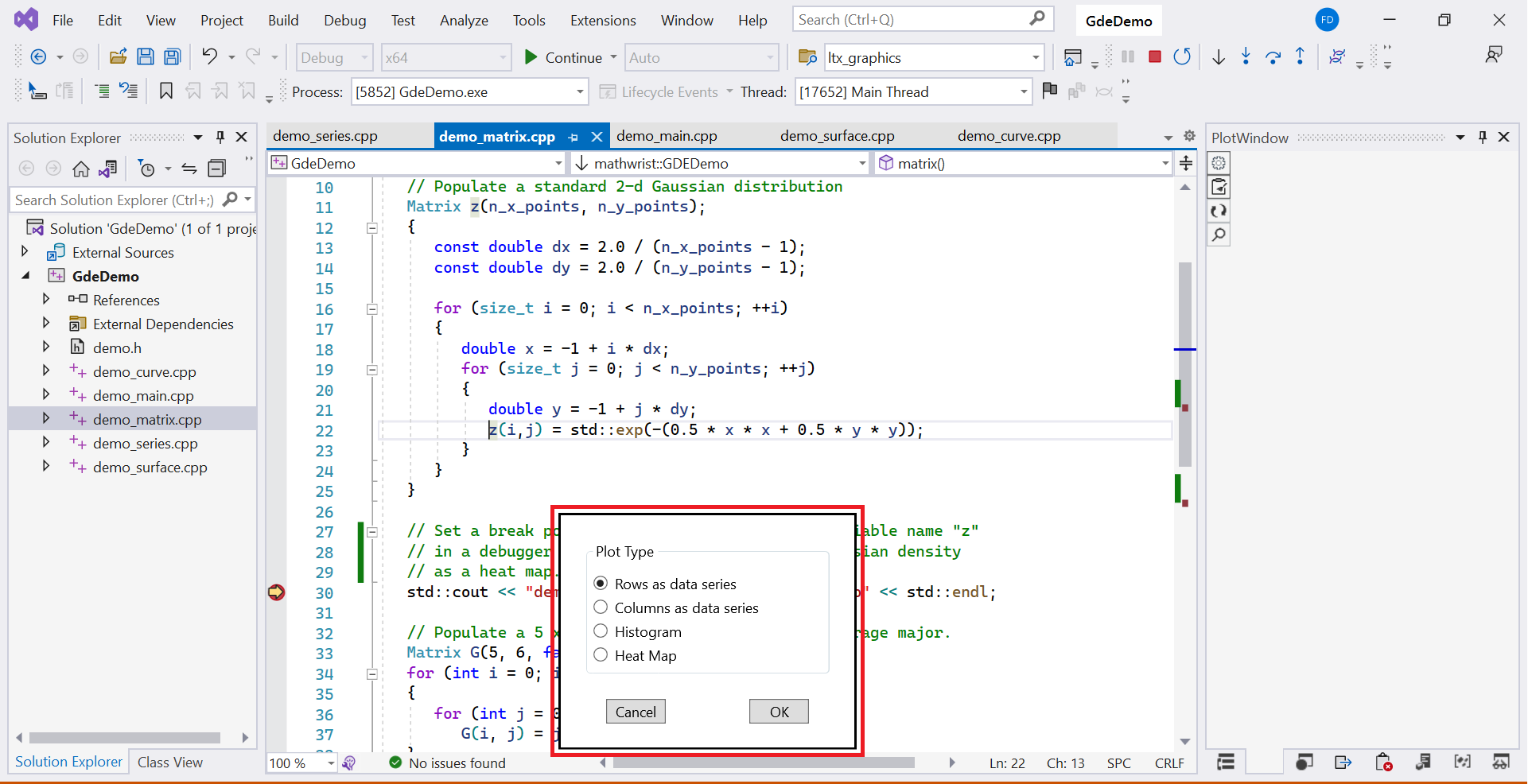
Since is a symmetric matrix, plotting row series or column series does not matter in this example. But in general, this display orientation choice is remembered in GDE’s data session. Figure 6 illustrates the data series plot of matrix . Figure 7 is the final display of plotting matrix in heat map format.
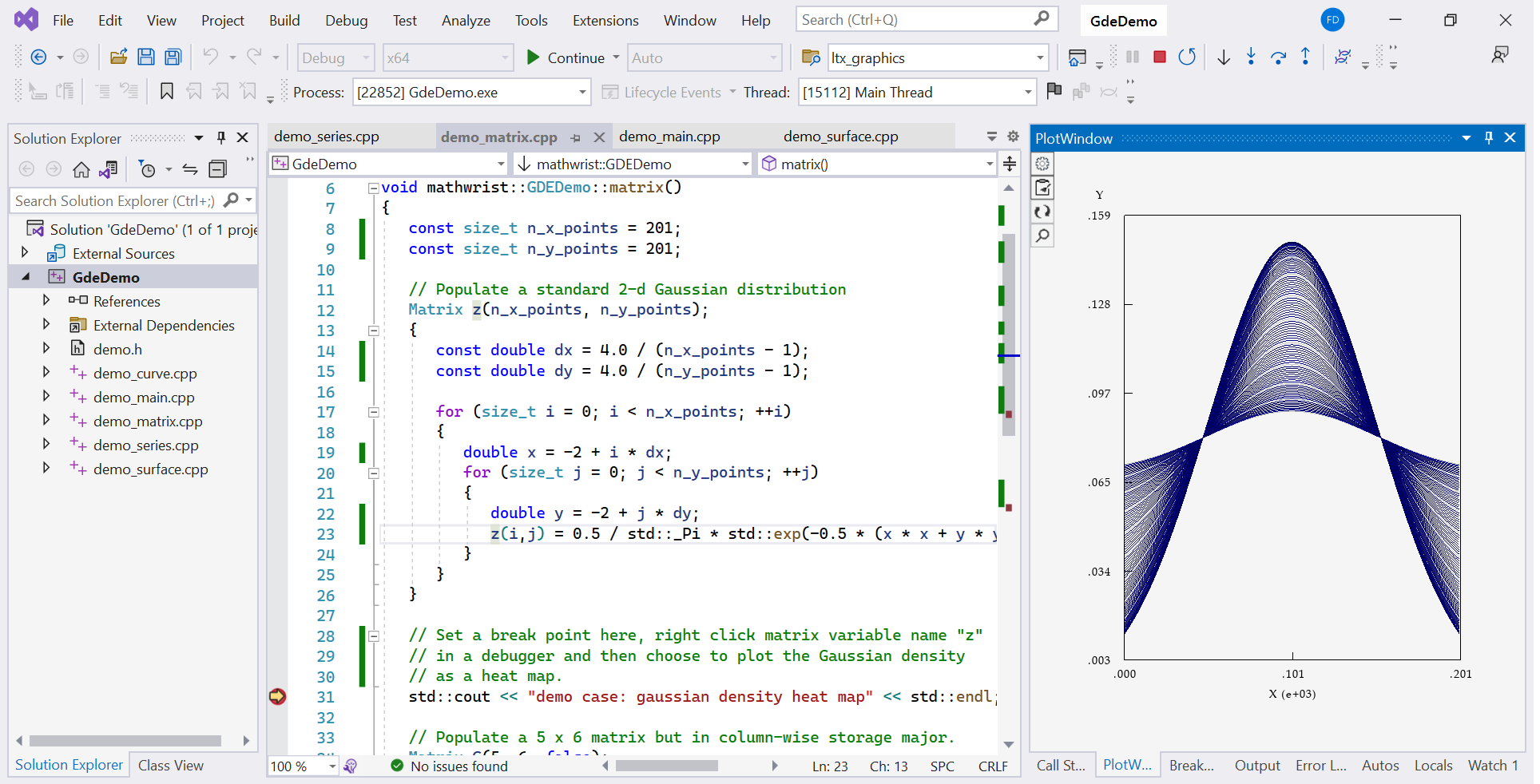
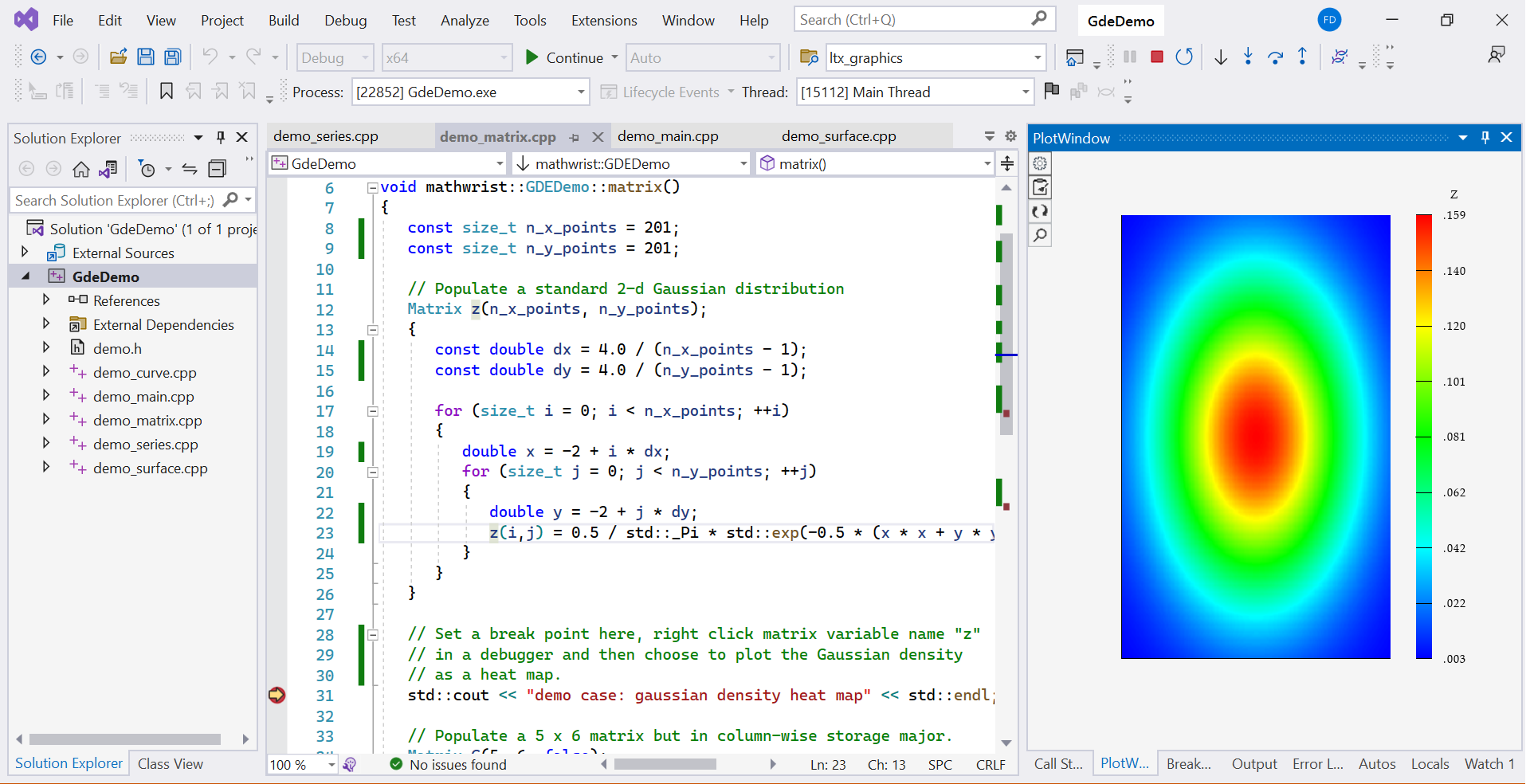
3 Session Management
After we plot signals and sp_returns in example Listing 1, these two vector type objects are added to the “Arrays/Vectors” section of GDE data session. We can start the demo program again with command line argument –matrix and plot matrix object , which is added to the “Matrices” section on GDE data session. Clicking the “Plot Settings” button , we can see the “Data Session” tab illustrated by Figure 8.
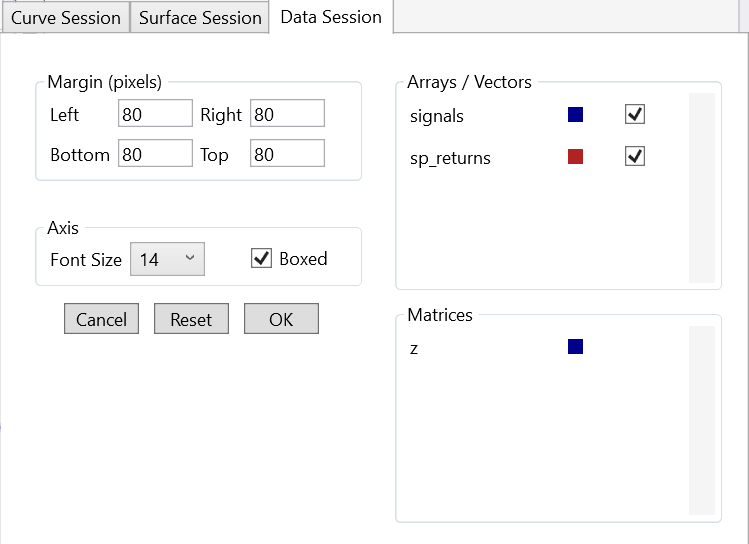
Users can then customize the display using the following UI controls at the left of the tab.
-
•
Margin, set the display margin in the unit of pixels around the plotting area.
-
•
Font size, set the text font size on the data plot.
-
•
Boxed, if selected, the plot area will display a solid line at each border.
-
•
Canel, cancel the display setting change.
-
•
Reset, reset the settings back to default values.
-
•
OK, plot the data session using the current customized settings.
On the right side of the data session tab, the top “Arrays/Vectors” section lists array/vector like plottable components. The bottom part lists matrix plottable components. In this example, we see the buffer object signals is recognized as “array/vector” type. One-column matrix object sp_return is treated as a vector and appears in “Arrays/Vectors” section as well. Matrix object on the other hand appears in the bottom section.
The “Arrays/Vectors” section has three columns, variable name, color coding and a checkbox. Right click on a variable name, a context menu appears as the red box area in Figure 9. Menu item “Histogram” and “Series” allow users to plot this component as a standalone histogram or data series. Menu item “Delete” will remove this component from the data session. The checkbox column is used to select multiple vector components and plot them together in the same data series display after clicking the OK button.
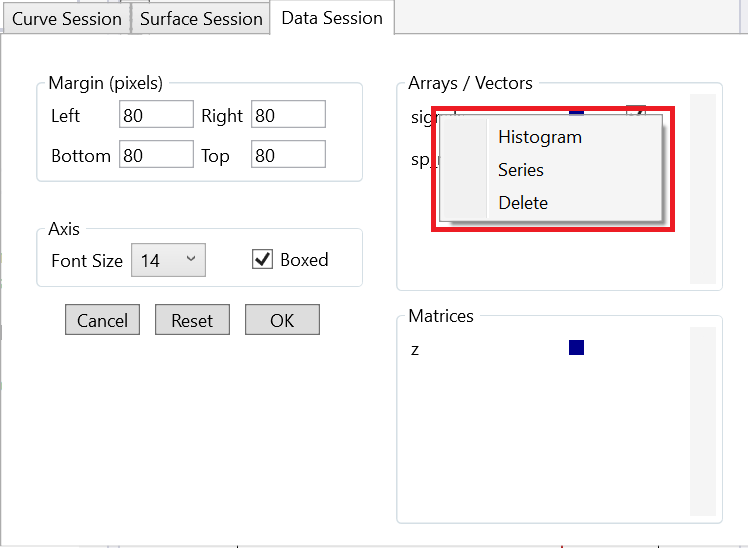
The “Matrices” section has only component name and color coding two columns, since matrix objects are always displayed separate from each other. Right click a component name brings up a context menu illustrated as the red box in Figure 10. Matrix objects can be plotted as histogram, series or heat map from the context menu. “Delete” command will remove a matrix plottable from the data session.
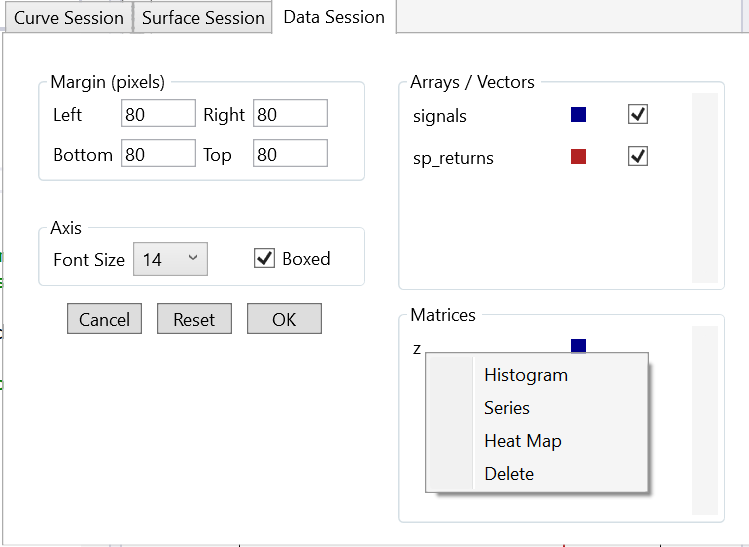
4 NPL Plottable Types
Mathwrist’s own buffer and matrix classes, mathwrist::ftl::Bufferdouble and mathwrist::Matrix, are treated the same way as user-defined vector and matrix plottable types. In addition, direct view objects from mathwrist::Matrix::View and mathwrist::Matrix::ViewConst classes are understood as matrix plottable as well. Here, a direct matrix view means that the view object directly mapps to some contiguous memory. Indirect matrix views, i.e. transposed view, matrix sub views, permutated views etc., are not supported as plottable types.