GDE Curve Plots
Mathwrist User Guide Series
Abstract
This document is a user guide of using Mathwrist’s Graphical Debugging Extension (GDE) tool to visualize curves, 2-d scatter data points and NPL math objects in Microsoft Visual Studio IDE. NPL is Mathwrist’s C++ numerical programming library. 2-d functions defined in NPL are directly recognized as plottable types in GDE. Please refer to our NPL documentation series for details. For an overview of GDE, please refer to our “GDE Overview” user guide.
1 Introduction
In this document, we focus on the GDE “Curve Session” and give details on plotting 2-d scatter data points, imposing a smooth curve fit to these data points and directly visualizing NPL 2-d math objects. It is recommended to first read our “GDE Overview” user guide in order to develop a high level understanding of GDE.
When an object is of a user defined matrix data type that has been exported to GDE as a part of plottable specification json file, GDE will further check if this matrix object has exactly 2 rows or 2 columns. If so, GDE will provide a choice to plot the columns of a 2-row matrix or the rows of a 2-column matrix as 2-d scatter data points. In addition, user may choose to plot the 2-d scatter pointer together with a smooth curve fit. We will illustate this feature in the next section by a walkthrough example.
When NPL is used in a user’s application, NPL matrix class mathwrist::Matrix, matrix views mathwrist::Matrix::View and mathwrist::Matrix::ViewConst are recognized as user-defined matrix types. Therefore 2-row or 2-column matrix objects and direct view objects can be plotted as 2-d scatter data points. Moreover, many concrete 2-d function and curve types defined in NPL can be added to GDE curve session for plotting.
2 Walkthrough Example
In this example code Listing 1, we populated some artificial data points from line 10 to 33, where , for some random noise . We store these data points to a 2-column matrix sin_x_pi from line 35 to 41. Here sin_x_pi is a user-defined matrix type mathwrist::GDEDemo::Matrix, which we have exported as a consecutive memory matrix in our “mathwrist.gde.spec.json” file.
After the data points are saved to sin_x_pi, we set a break point at line 45 and launch a debugging session. Right click variable sin_x_pi and choose “plot sin_x_pi” in context menu commands. Because sin_x_pi is a 2-column matrix, each row can be interpreted as a pair of data point. GDE will pop up a display choice window that includes “Rows as scatter points” and “Rows as scatter points (fit)” as illustrated in the red rectangle of Figure 1.
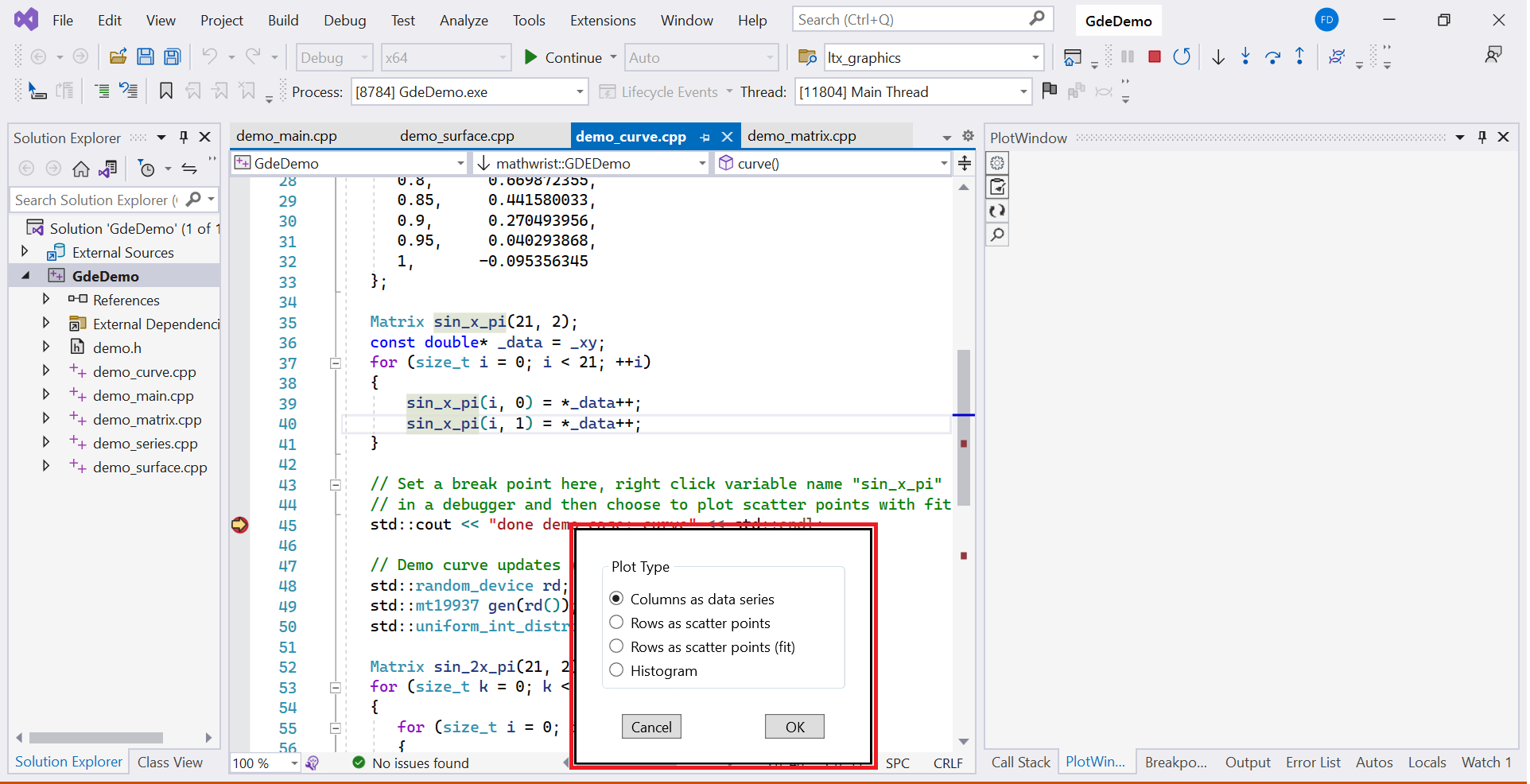
The left plot 2(a) in Figure 2 displays 21 scatter points corresponding to the radio button choice “Rows as scatter points”. The right plot 2(b) in Figure 2 is the result of choosing “Rows as scatter points (fit)”, which displays a smooth curve fitted to those scatter points.
![]() |
![]() |
Lastly, the code block from line 52 to 63 populates another set of scatter data points from function and saves them to matrix object sin_2x_pi. We can add this object to the same plot and display it together with sin_x_pi as Figure 3.
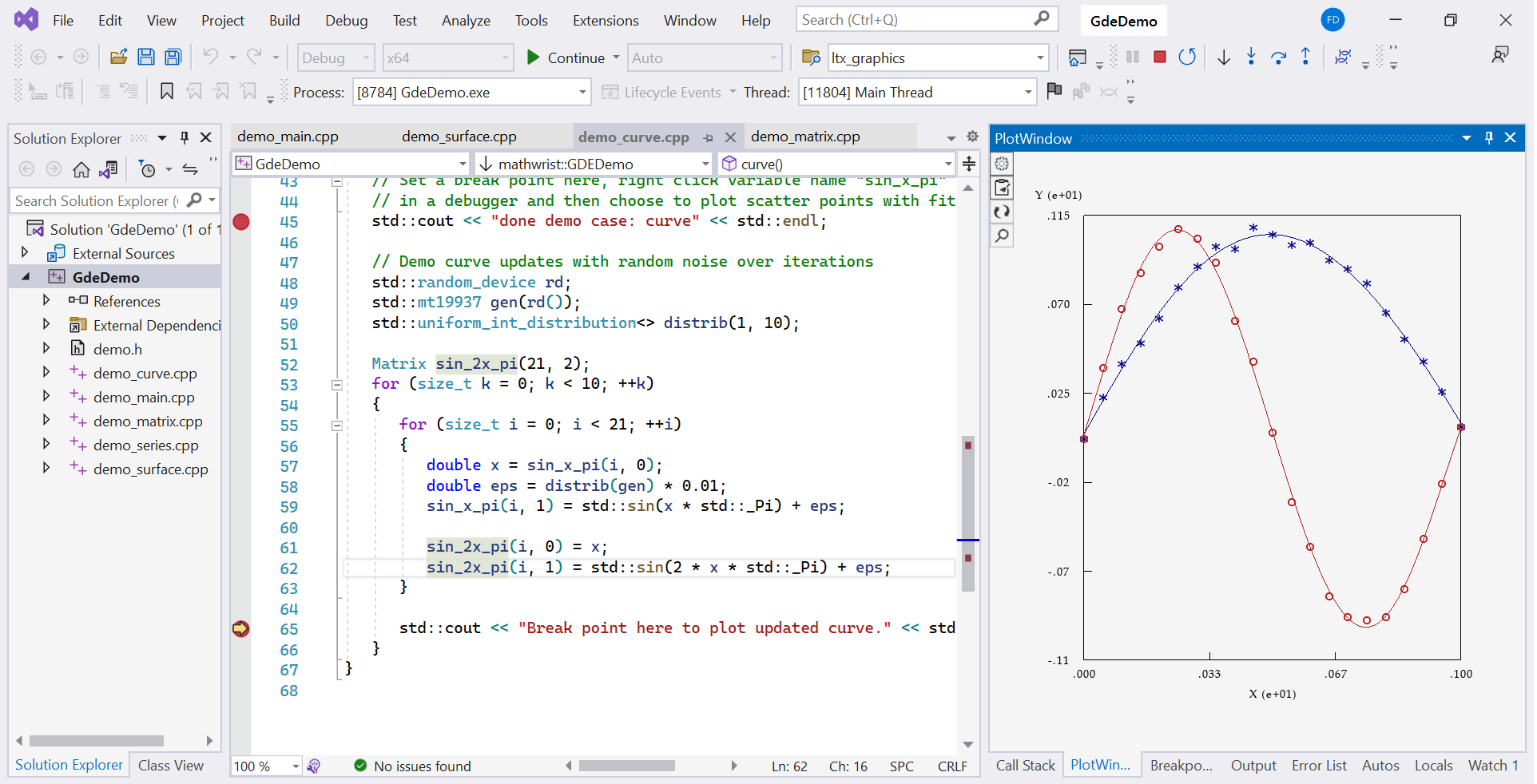
Note that we have adjusted the range of coordinate in display settings so that both sin_x_pi and sin_2x_pi fit in the same plot. Further, setting a break point i.e. at line 62, we can watch how these two objects are updated by random noise over iterations.
3 Session Management
Under the “Curve Session” tab in Figure 4, users can customize the display using the following UI controls.
-
•
Margin, set the display margin in the unit of pixels around the plotting area.
-
•
Axis min/max, set the axis range that function is plotted.
-
•
Axis min/max, set the axis range that function is plotted.
-
•
Font size, set the text font size on the curve plot.
-
•
Boxed, if selected, the plot area will display a solid line at each border.
-
•
Cancel, cancel the setting change.
-
•
Reset, reset the settings back to default values.
-
•
OK, plot the curve session using the current customized settings.
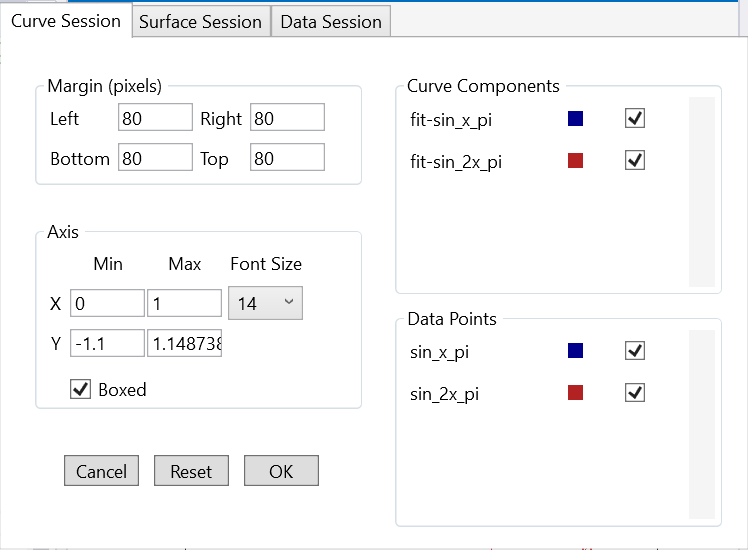
The right part of Figure 4 lists all curve components and scatter data points that have been added to the curve session. This component list has 3 columns, component name, color coding and selection checkbox. Note that the curve fit component name has a prefix “fit-” added to the variable name.
The checkbox control in component list section allows users to select which component is to be displayed together in the same plot. Check a curve fit component will automatically include its source data point component for display. Reversely, uncheck a scatter point component will automatically exclude its curve fit component from the display. Right clicking an individual component’s name will trigger a context menu “Delete” to delete this component from the curve session.
4 NPL Plottable Types
The following NPL C++ classes are recognized as 2-d plottables and can be added to GDE curve session for plotting.
-
•
mathwrist::FunctPiecewiseConst, a piecewise constant function.
-
•
mathwrist::FunctPiecewiseLinear, a piecewise linear function.
-
•
mathwrist::FunctPiecewiseLinearJump, a piecewise linear function that jumps at knot points.
-
•
mathwrist::BSplineCurve, a smooth curve that uses B-spline as basis functions.
-
•
mathwrist::InterpCubicSplineCurve, a cubic spline interpolation curve.
-
•
mathwrist::ChebyshevCurve, a smooth curve that uses Chebyshev polynomial as basis functions.
-
•
mathwrist::Matrix, mathwrist::Matrix::View and mathwrist::Matrix::ViewConst, a matrix or a direct matrix view that has 2 rows or 2-columns.
Figure 5 is an example screen shot of GDE curve plot in NPL’s demo project, where we have fitted a smooth mathwrist::BSplineCurve object. Right click the variable name spline of this object in Visual Studio debug session, and then click “plot spline” from debugging context menu. GDE then plots the curve in plot window at the right side of the IDE.
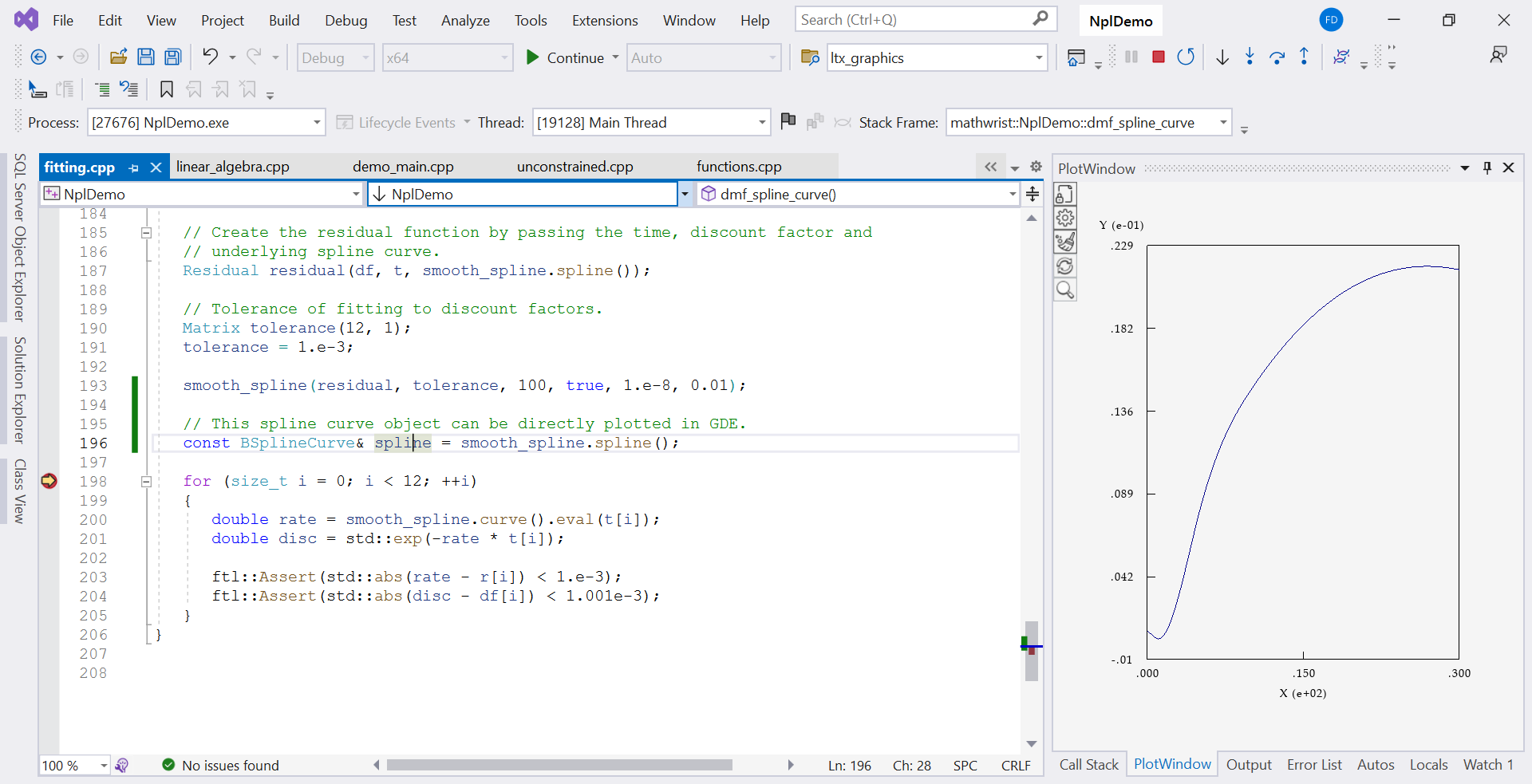