User Guide for Mathwrist’s Numerical Programming Library
Abstract
Mathwrist’s Numerical Programming Library (NPL) is a C++ dynamic linked library (dll) that provides numerical programming tools for scientists and engineers. The library is designed to integrate mathematical concepts, operations and numerical algorithms in an object-oriented approach. Users are expected to have intermediate level C++ programming knowledge and experience. This document is a general guideline to install and use NPL.
1 Installation
Mathwrist uses a standard Windows software installer to deploy the C++ Numerical Programming Library (NPL) product together with a license activation application to user’s machine. The target installation machine is required to have the following configuration in order to run the software:
-
•
x64-based processors;
-
•
64-bit Microsoft Windows operating system11 1 Please contact us at sales@mathwrist.com for licensing and software availability on other platforms.;
-
•
Microsoft .NET framework 4.822 2 .NET framework 4.8 is a recommended Windows update and generally is installed automatically on Windows platform.
Our software installer is available at Microsoft Store. Double click the installer program and follow the standard Windows software setup steps as illustrated in Figure 1.
![]() |
![]() |
At the “Setup Choice” step in Figure 1(b), it is recommended to use the default installation directory “C:Program FilesMathwrist” and install the software for everyone. This requires local admin right on the target machine. After the software is successfully installed, users can find “Mathwrist Activation and NPL” appears in Control PanelProgramsPrograms and Features. Users can also find the “Mathwrist” folder from Windows Start Menu that has a menu item for license activation.
2 License Activation
Mathwrist’s Graphical Debugging Extension (GDE) and NPL products use the same license activation application to connect to Mathwrist’s software licensing server and generate a node-locked license file. Users may need adjust firewall setting to allow the activation application to connect to Internet.
To launch the license activation process, please go to Windows start menu Start MenuMathwristLicense Activation. After the license activation application is started, users have the opportunity to acknowledge and accept the “End User License Agreement (EULA)” terms and conditions as illustrated in Figure 2. In the installation directory, users can find a copy of EULA text file “eula-mathwrist.txt”. Mathwrist reserves all rights to update its EULA terms at software distributions.
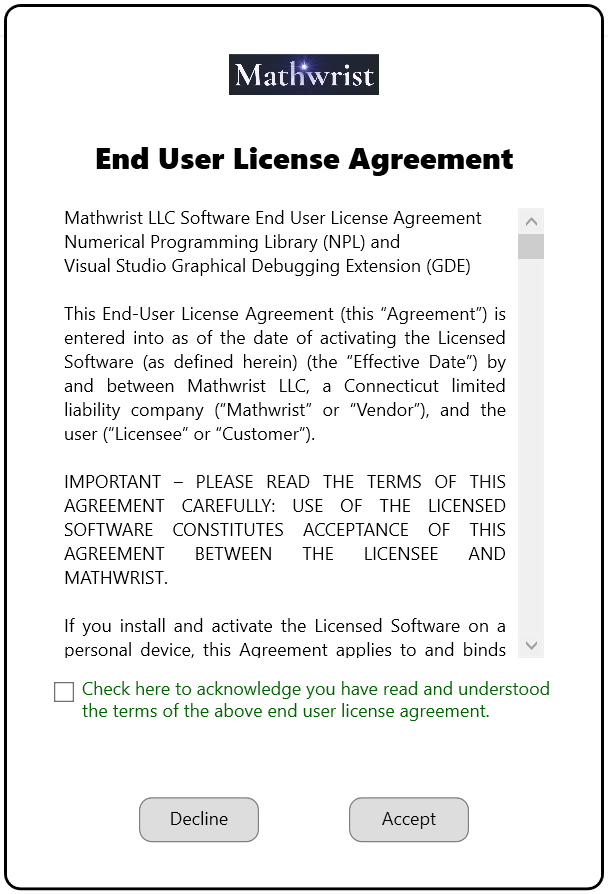
After users accept the EULA terms and conditions, the activation application then proceeds to the next step illustrated in Figure 3. Please click all checkboxes and the next button to generate a 12-month software license to use both GDE and NPL free of charge. At the last step upon successful activation, shown in Figure 4, users need save the license file to Mathwrist installation directory. This also requires write permission to Mathwrist directory.
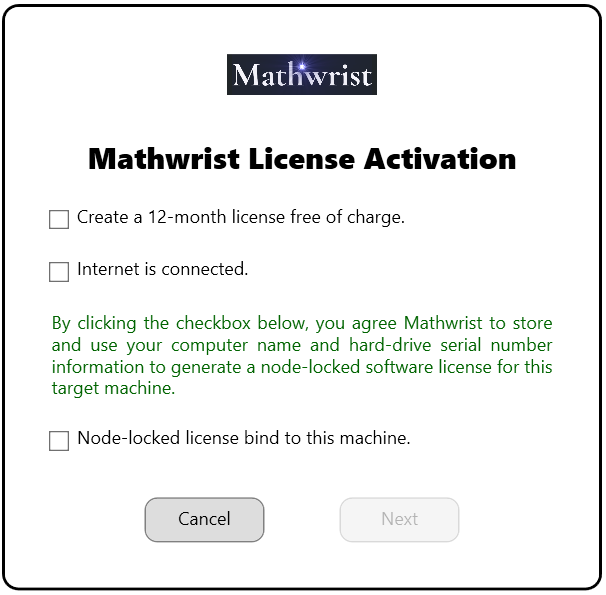
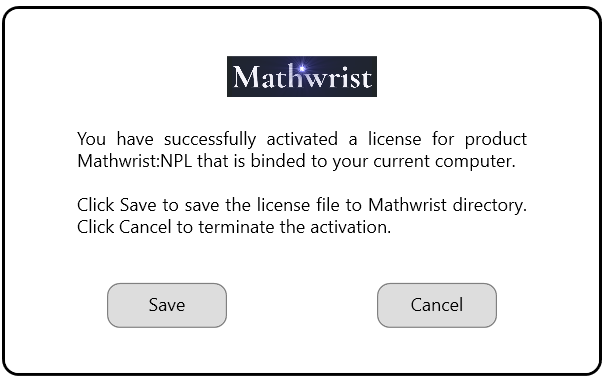
3 Environment Setup
3.1 Environment Variable
Our license activation application automatically creates an environment variable Mathwrist that points to Mathwrist software installation directory. At run time, NPL searches for a valid license file under the path set be this environment variable.
3.2 Installation Directory
Under Mathwrist installation directory, default to “C:Program FilesMathwrist”, users can find the following subdirectores:
-
•
activation: contains binary executable files to activate a node-locked software license.
-
•
demo: contains C++ demo projects to use NPL and GDE.
-
•
doc: containts NPL and GDE documentation pdf files.
-
•
include: is the root directory of NPL C++ include header files.
-
•
lib: contains C++ lib files to link to NPL.
NPL C++ header files are stored in “ftl” and “npl” subdirectories under the “include” root directory. When creating a Visual Studio C++ project, users need set the project properties C++GeneralAdditional Include Directories to this “include” root directory.
There are to two C++ lib files “MathwristNPL-D.lib” and “MathwristNPL.lib” under the “lib” directory. They are the 64-bit lib files to link NPL in debug build and release build respectively. Users need add a path to this lib directory in C++ project properties LinkerGeneralAdditional Library Directories.
Users also need set debug and release build properties as the following:
-
•
Debug build:
-
–
C/C++Code Generation: Multi-threaded Debug DLL (/MDd)
-
–
LinkerInputAdditional Dependencies: add MathwristNPL-D.lib
-
–
-
•
Release build:
-
–
C/C++Code Generation: Multi-threaded DLL (/MD)
-
–
LinkerInputAdditional Dependencies: add MathwristNPL.lib.
-
–
The run time dlls “MathwristNPL-D.dll” and “MathwristNPL.dll” are directly stored under Mathwrist installation directory. Users need add a path to this directory in their environment variable PATH settings in order to load NPL dlls at run time.
4 FTL
Mathwrist has its own C++ “Fundamental Template Library” (ftl), which is a lightweight implementation of some utility tools to facilitate internal software development. The goal of ftl is to avoid over-generalization and reduce the footprint of templates in NPL.
The _cloneable.h and _iterator.h two headers under “includeftl” directory are used internally for NPL implementation only. They are not involved at NPL API level hence not documented.
4.1 _exception.h
This header defines a C++ class mathwrist::ftl::Exception. NPL may throw exception objects of this class for various causes in the library. In general, users need not concern about how to create objects from this class. The following code illustrates catching exceptions thrown from NPL and retrieving error messages or error codes.
4.2 _buffer.h
This header file defines a dynamically allocated buffer class mathwrist::ftl::Buffer<T> for template type T. At API level, NPL could take const ftl::Buffer<T>& as input argument or ftl::Buffer<T>& as output argument. GDE automatically recognizes a ftl::Buffer<double> object as a vector-type plottable object.
The public functions of this buffer class are documented in place in the _buffer.h header file. Please note that the buffer class does NOT perform index range checks. It is user’s responsibility to ensure a buffer object has correct size and index access.
5 Programming with NPL
5.1 Predefined Constants
NPL uses a set of predefined double constants, hardcoded in header file “constants.h”. Some of them are real mathematical constants. Some of them on the other hand serve as practically decent numerical default values. It is not our interest to debate what are “truly” constants, what are the “true” values on certain platform architect and why some C++ std numeric limits are not used. Users are welcome to choose appropriate alternatives in their applications unless explicitly stated in NPL documentation.
5.2 C++ Raw Pointers
NPL API accepts C++ raw pointers as arguments. Whenever NPL caches user provided raw pointers as class data members, i.e. the and pointers to create piecewise functions, our API documentation will explicitly state this situation. It is required that the underlying memory of these pointers is valid during the entire lifecycle of the pointer-holding object.
Typically, NPL API functions take a C++ raw pointer together with a size argument that specifies the number of elements allocated in the pointed memory. However when the context has no ambiguity regarding the size, NPL API might simply take the pointer alone as the following example.
In this example, a BSplineCurve object consists of certain number of B-spline basis. The number of basis coefficients provided through the pointer argument _beta is assumed exactly equal to the number of basis stored in the BSplineCurve object. The query function n_basis() can retrieve the number of basis. In such situations, it is user’s responsibility to ensure pointer _beta has correct number of basis coefficients.
As a general principle, when NPL receives input from raw pointers provided by users, it is users’ responsibility to manage the memory of their pointers. If NPL provides a pointer to users, the pointer associated memory is managed by NPL.
5.3 Matrix Arguments in User Defined Functions
In various places, e.g. the objective function for numerical optimization, users need supply a user-defined function that is derived from the FunctionND interface. This n-d function interface takes Matrix objects as input and output arguments. As a convention, it is ensured that whenever a user-defined function is called inside the library, NPL always passes matrix arguments that have all elements stored in contiguous memory. These matrix arguments support iterator access. It is also legitimate to obtain raw pointers from these matrix objects, illustrated as the code example.
5.4 Numerical Range
NPL uses Bound class to define a numerical range. A numerical value is considered as a bounded quantity. The lower bound and upper bound parameters provided to Bound class constructor need be strictly in . In addition, NPL assumes . A quantity exceeds the “infinity” value typically suggests numerical noise. It is a good practice to formulate mathematical problems into numerically normalized problems, i.e. properly scaled.
When we compare double quantity to , NPL uses to test the equality condition, where usually is a tolerance control parameter passed down from API level functions. In occasional cases when the equality comparison involves “exactness”, i.e. test if a knot point is hit, NPL takes , where is the “machine epsilon” defined in constants.h. In all other cases and when tolerance control is not specified, we use default value , where is the “numerical epsilon” defined constants.h.
5.5 Thread Safety
NPL internally does not apply any threading or concurrency protection. It could be considered as “thread safe” in the sense that there is no writable local static data being used inside the library. Further, MathwristNPL-D.dll and MathwristNPL.dll are built with Microsoft multithreaded runtime libraries. So NPL can be used in a multithreading environment.
In general however, concurrent access to the same NPL object from different threads should be avoided unless they are simple query functions. In particular, NPL math objects may hold mutable resources to facilitate efficienct calculation. So a C++ const member function does not necessarily imply “read only” access in NPL. As a general principle, calling computing routines on a shared object from different threads should be avoided.